I’ve been waiting for this for a long time! Because today we can start again with a set of JEPs, which we will probably see around JDK 22. In addition, Pulumi, and interesting releases. And finally – an invitation!
1. First set of new JEPs following the release of JDK 21.
JEP 455: Primitive types in Patterns, instanceof, and switch (Preview)
if (json instanceof JsonObject(var map)
&& map.get("age") instanceof JsonNumber(double age))
{
return new Customer(name, (int)age);
}
if (json instanceof JsonObject(var map)
&& map.get("name") instanceof JsonString(String name)
&& map.get("age") instanceof JsonNumber(int age))
{
return new Customer(name, age); // bez konwersji!
}
JEP 458: Launch Multi-File Source-Code Programs
JEP 457: Class-File API (Preview)
Additionally, JEP 456: Unnamed Variables and Patterns has also been released, which basically unchanged stabilises JEP 443, known from JDK 21.
It’s also quite fascinating to delve into Draft Valhalla, which discusses Null-Restricted Value Class Types (!). However, I plan to take a measured approach to this – I believe we’ll dedicate an entire separate section to it once it becomes more stable.
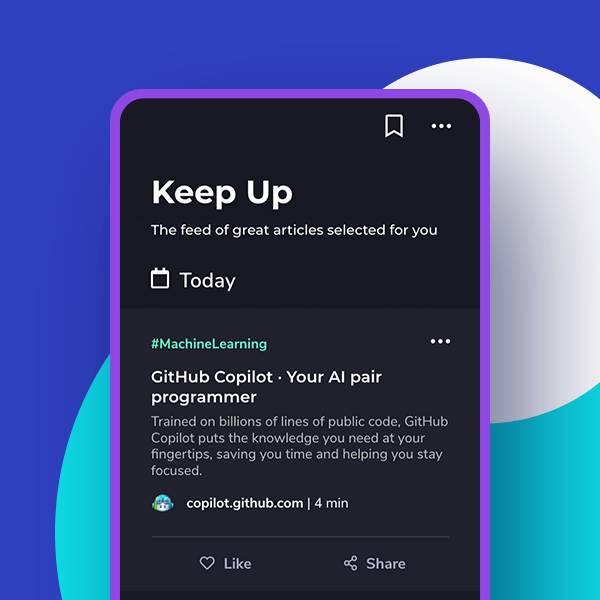
2. Pulumi – Infrastructure-as-a-Code in Java, Kotlin and Scala
And now things are about to get untypical as we delve into the topic of Pulumi. But don’t fret, I have a solid reason for this. Unfamiliar with Pulumi? I understand that you’re not currently reading “Infrastructure as a Code Weekly”, so let’s begin with a quick overview. In my opinion, the simplest method to explain Pulumi is by comparing it with its main rival, Terraform.
For individuals without experience in DevOps, infrastructure, and other cloud-related aspects (and a small piece of advice – if you don’t have a very precise career path, you should gain one), Terraform is a tool that manages infrastructure similarly to other code. However, it’s not exactly the same “code”, as Terraform employs a specialized HCL language to declaratively describe resources in the cloud. The idea is that users specify their desired infrastructure, and Terraform ensures this state is achieved with providers for different cloud platforms – all in a fully declarative manner.
However, as programmers (right?), it’s time to consider the competition. Pulumi, the main hero of this section, is similar to Terraform in that it’s used to manage infrastructure as code. However, it has a significant difference: it permits the use of standard programming languages (like Python, TypeScript) instead of a dedicated language. This enables Pulumi users to utilize loops, conditions, and other language features when defining infrastructure, providing additional flexibility over Terraform. This becomes incredibly beneficial when attempting to perform slightly more ‘intelligent’ tasks in our infrastructure than merely piecing together a few yamls from the internet.
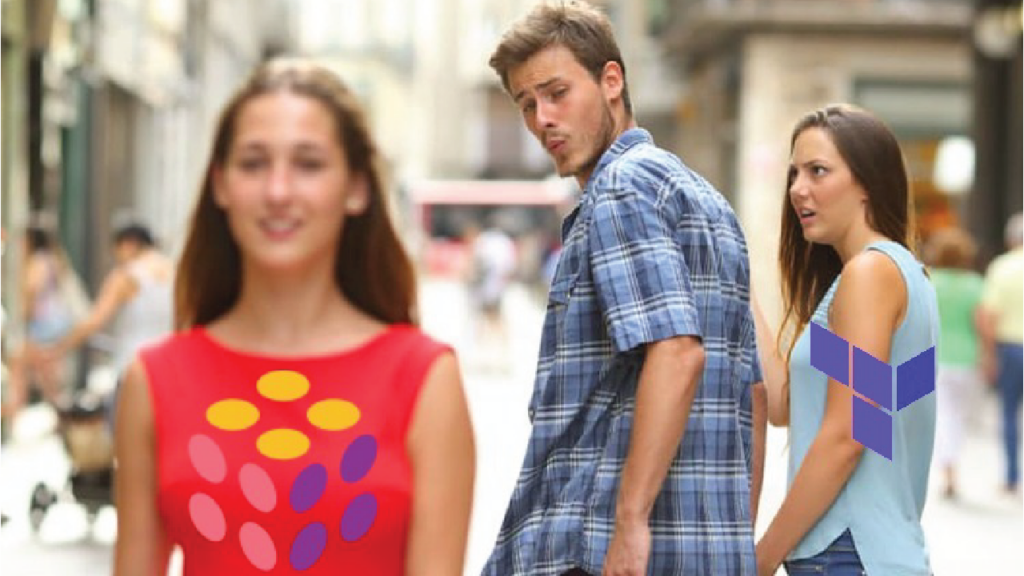
Last week, Pulumi secured a significant amount of funding to further develop its tools. This happened during a period when Venture Capital is not as generous as before, indicating the potential of the tool. This event coincided with Terraforma’s licensing problems, which you can find more details about here. This news prompted me to discuss the JVM versions of the Pulumi SDK, particularly since engineers from my main company, VirtusLab, played a significant role. As a business, we are deeply embedded in the open-source and JVM tooling universe, accountable for the Scala compiler among other things. Believing in Pulumi’s vision, we collaborated with them to create an official Java version and variants in Scala and Kotlin.
This latest release holds a special place for me. I firmly believe that the future will see the realization of the Kotlin Multiplatform vision, where a single language can be used to create all project ‘artifacts’ – the whole package. It’s no surprise that application code can be written in Kotlin, but JetBrains is also deeply involved in Compose – the UI layer – and Gradle, which will be discussed later today, is also deeply committed to the Kotlin DSL. Infrastructure has been neglected, and Terraform with its HCL is causing significant disruption. This is why we ensured the creation of Pulumi for Kotlin. Julia Plewa, in particular, contributed significantly and she shared the project’s vision and the “first steps” in the article Pulumi Kotlin – The missing piece in Kotlin multi-platform, which perfectly encapsulates the entire project’s vision.
Besom, the Scala SDK for Pulumi, shares a similar philosophy. The developers’ goal is to enable developers to realistically create ‘full-stack’ applications in their preferred language, without the hassle of dealing with yams. The project can be found on Github. Additionally, here’s a link to a tweet announcing the project, which effectively highlights the philosophies behind it.
Do you agree with this method? If yes, give Pulumi a shot – it’s been quite a while since I, for one, enjoyed writing “infra” this much.
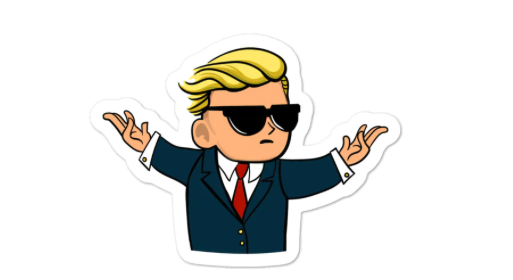
3. Release Radar
JVector
Vector databases are an expanding area in the database realm and the broader IT community, due to their application in LLMs. In fact, vector search is crucial in contemporary applications that utilize generative AI, simplifying the process for developers to augment the knowledge base of models with extra data. This enables sophisticated language models to deliver accurate responses, preventing mistakes or ‘hallucinations’.
It’s not unexpected to see comparable technologies being developed in Java. I believe many readers already know that a number of database solutions rely on the JVM. These encompass Apache Cassandra, HBase – a columnar storage for the Hadoop file system, and Elasticsearch, a search and analysis engine built on Lucene.
JVector is a vector search engine that can be embedded, written entirely in Java. It powers DataStax Astra and integrates with Apache Cassandra, as previously mentioned. JVector’s closest counterpart is the vector search of Apache Lucene. Lucene does implement the HNSW vector search algorithm, which is speedy but tends to be memory-hungry. JVector, on the other hand, is based on the more advanced DiskANN algorithm, making it over 10 times faster than Lucene when dealing with large datasets. JVector is quick, memory-efficient, disk-aware, parallel, easy to embed, and incremental. The JVector project aims for easy integration while still maintaining high performance. For instance, it utilizes the Vector API and SIMD instructions from Panama (which, for clarity, is still in incubation).
Gradle 8.4
The Gradle team has introduced version 8.4. The most significant update is the compilation support for JDK 21, and the embedded Kotlin has been upgraded to version 1.9.10. Interestingly, Kotlin 1.9.10 does not yet support JDK 21, implying that Gradle requires JDK 20 to function.
In this release, Windows also got the Java compiler daemons-based acceleration that other systems received in version 8.3. The update includes minor enhancements like optimized memory settings for code quality control tools (such as Checkstyle, CodeNarc, and PMD) in larger codebases, a better Checkstyle HTML report format, and compatibility with the JVM distributed by JetBrains.
Also a significant modification is the easier creation of role-focused configurations, with anticipated support for basic Gradle plug-ins for use as soon as the forthcoming version 9.0. The Kotlin DSL has also been improved, with stable support for simple property assignment using the =
operator.
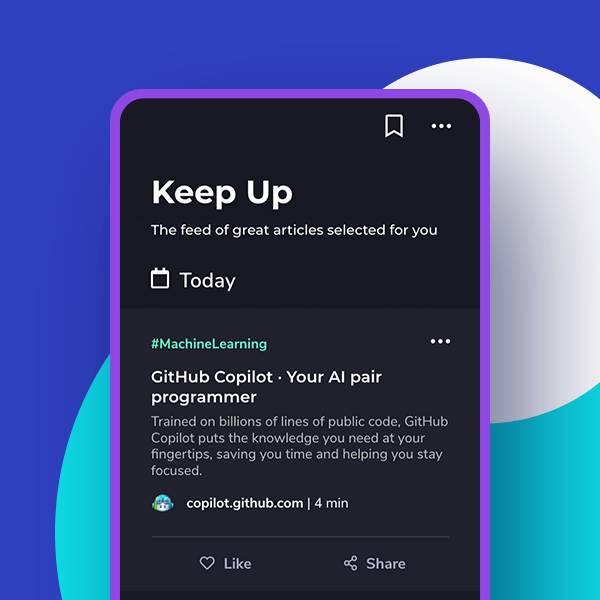
Bonus: Small invitation.
And finally, if you reside in Prague or the Stuttgart vicinity, I would like to extend an invitation for you to meet me in person. Considering my upcoming week is somewhat hectic (which might lead to the absence of an edition next week, but I’ll strive!).
First, I’m heading to Ludwigsburg, Germany for EclipseCon, where I’ll be presenting GraalVM, CRaC, Leyden and friends – in search of TRULY cloud-native Java on the 19th of October.
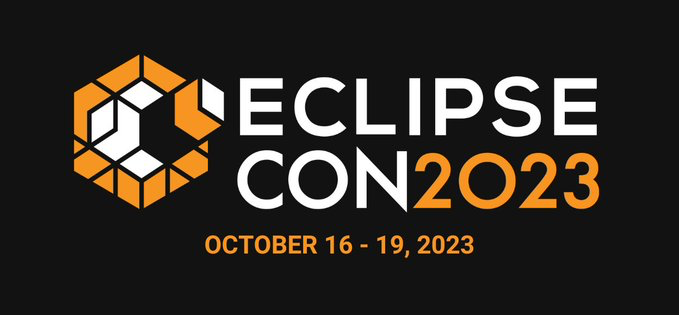
Then a swift teleportation (since I’m unsure of what else to label it) to Prague 🇨🇿 to attend GeeCON on 20 October.
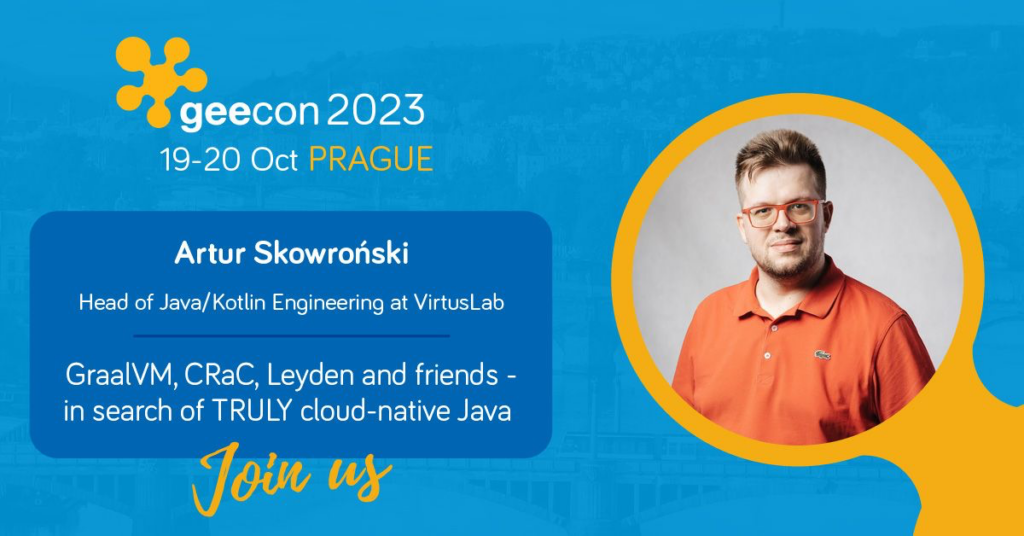
This is how we roll 😎
Even if you’re not attending the conferences, if anyone is interested in meeting up for a coffee, I believe we can arrange it. If needed, you have my email.