Wondering what’s going on with React Server Components and what happened to the long-promised React Forget compiler? If so, you will find the answers to these questions in today’s report. Besides, you’ll find out what new features are heading to JavaScript and how Microsoft improved Teams by migrating away from Electron to WebView2
1. what is the React team working on?
This week, React team shared with us a summary of all the initiatives taking place in the project. The note really isn’t long, so I recommend everyone to read it. Meanwhile, for everyone too busy with pre-Easter preparations, we have a brief summary of the most important things.
- React Server Components is a new architecture that React team has been working on since 2020. They are still in beta, but are currently the team’s top priority. We can already use React Server Components in Next.js or Gatsby. If you want to catch up on React Server Components, the best source would be the article published on the Plasmic blog “How React server components work: an in-depth guide”.
- Since the publication of the last update, the convention for labeling server and client components has changed. Instead of the
.server.tsx
and.client.tsx
extensions, we will use the shebanguse client
anduse server
. - Work has also moved forward on an RFC on async/await support. Server components will be able to use JavaScript syntax directly, while client components will be able to unpack Promises using the new
use(Promise)
hook. You can read more about how the new hook will work in the 109th edition of our review. - The biggest news regarding React Server Components are Server Actions. These will enable client components to perform operations directly on the server. We can expect a detailed RFC soon.
- The team is currently working on integrating the
<Suspense />
component with browser resource loading such as CSS files and external scripts. We can expect a detailed RFC very soon. - Another functionality the team is currently working on is support for
<title>
and<meta>
tags. Currently there are existing solutions to manipulate these parameters (e.g. Helmet), but they require rendering the entire application on the server. Such behavior conflicts with the idea of React Server Components, and for this reason the team decided to prepare a special API built into the library. We can also expect a detailed RFC soon. - React Forget is a compiler announced in 2021 that is supposed to take care of memonization (
useCallback
anduseMemo
) automatically. Work on the compiler has been significantly delayed, but it is already being used in the first projects inside Meta. As soon as the compiler starts working well enough, it will be made public. If you missed the topic of React Forget, the best source on it is still the original presentation of React without memo. - The last functionality mentioned in the note is Offscreen Rendering. The developers describe it as the equivalent of
content-visiblity: hidden
for React components. As the developers report, the first implementation is already being used by Meta in React Native, but we’ll have to wait a bit longer for a full-fledged RFC.
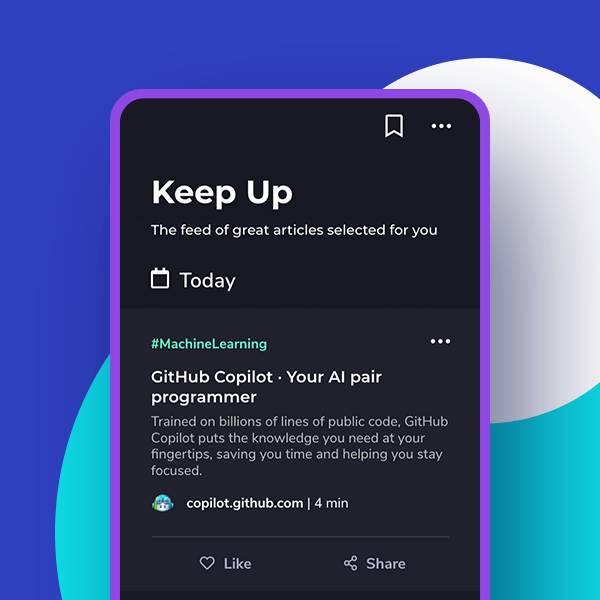
2 Elders are back in session – what interesting things came out of the next TC39 Meeting
This past week once again saw the TC39 Meeting, a gathering of JavaScript elders at which new features of the language are discussed. As usual, there were some interesting new features discussed, but before we get to the specifics, let’s briefly recap what TC39 is and what the standardization process looks like in detail.
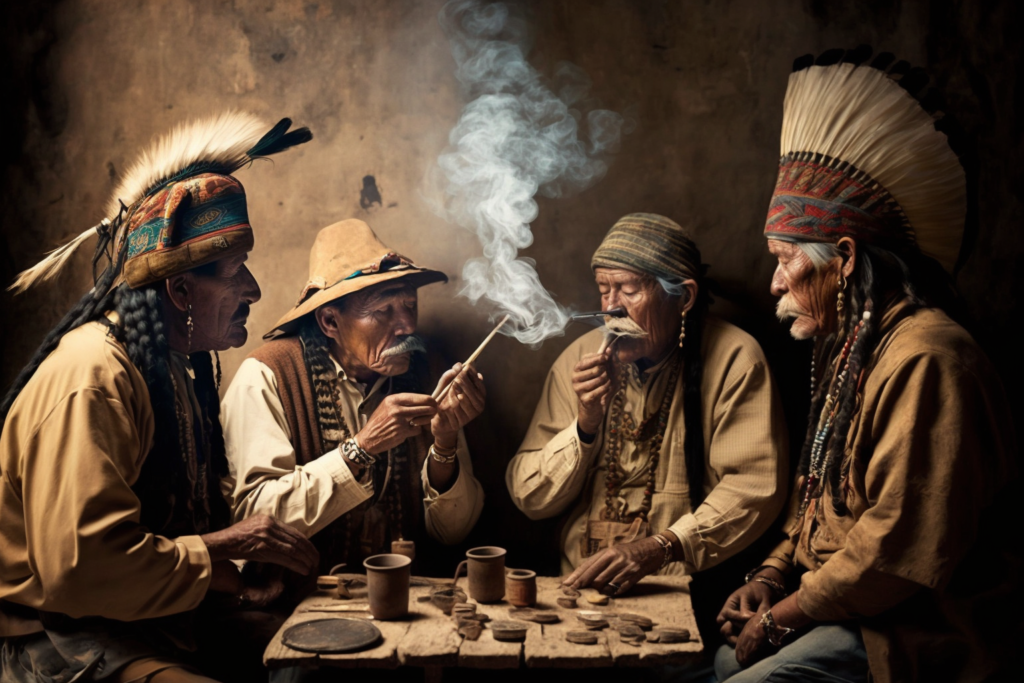
TC39 is a JavaScript standardization group made up of representatives of major stakeholders – such as the developers of Chrome, Safari, Firefox, Node and Babel. The process itself consists of 4 stages. Before a new functionality is presented at the TC39 meeting, a well-described Proposal is created and a so-called Champion is selected. Champion is the person responsible for the Proposal at all the stages of standardization. If a functionality makes it to the first stage, it means that the committee found the functionality interesting and wants to explore the topic further. When a functionality breaks through to the second stage, it means that it is already well thought out and the API is close to being finalized. After breaking through to the third stage, the API very rarely changes. At this stage, the first implementation and the necessary pollyfills are prepared. If the functionality makes it to stage four, it means that it will make it to the upcoming language specification, which is usually published in the first quarter of each year.
Below you will find a few selected features discussed at the last TC39 meeting that we found interesting. If you are interested in the full list, you can find it here.
Stage 3 – import assertions
It is widely recognized that if a functionality makes it to the third stage of standardization process, it is 90% likely to make it to the language standard soon. The import assertions functionality was in the unfortunate 10% and was moved back to stage two at the previous TC39 meeting. It is now back to Stage 3.
What’s the deal with import assertions? If this functionality makes its way into the language, we will be able to import JSON or WebAssembly files directly from within JavaScript. If this reminds you of anything, similar behavior can be achieved by properly configuring webpack.
import json from "./foo.json" with { type: "json" };
export val from './foo.js' with { type: "javascript" };
new Worker("foo.wasm", { type: "module", with: { type: "webassembly" } });
TC39 Proposal – Import Attributes
Stage 2 – Iterator.range()
Surely at some point you’ve faced the need to generate a list of numbers from a given range. Languages such as Python or Scala offer such functionality in the standard library. Unfortunately, until now JavaScrtipt was not equipped with such luxuries and we were doomed to either use external libraries (e.g. lodash or underscore) or some twisted hacks (a Stackoverflow thread suggests more than 20 possible solutions!). Fortunately, TC39 is already working on a solution to this problem. Interestingly, the new function will return an Tterator, which will allow us to operate on infinite sets!
// odd number from 1 to 99
[...Iterator.range(1, 100, 2)]
// numbers from 1 to 1000 that are divisible by 3
Iterator.range(0, Infinity)
.take(1000)
.filter((x) => !(x % 3))
.toArray();
// generator function yielding even numbers infinitely
function* even() {
for (const i of Iterator.range(0, Infinity)) if (i % 2 === 0) yield i
};
Stage 1 – Class method and consturcor decorators
Less than a few weeks ago, in Frontend Weekly vol. 120 we covered the history of decorators in TypeScript and JavaScript. This week a new Proposal on the subject has emerged. According to the current Proposal, which is in the third stage of standardization, only classes and methods can be decorated. This severely restricts framework developers, as parameter decoration is often used to provide additional context for Dependency Injection (e.g. @Optional()
or @Inject(TOKEN)
) or for automatic input validation (e.g. @ValidateEmail()
or @NotEmpty()
). The Proposal discussed at this meeting adds such a capability.
class UserManager {
createUser(@NotEmpty username, @NotEmpty password, @ValidateEmail emailAddress) { }
}
TC39 Proposal – Class method and consturcor decorators
Stage 1 – Promise.withResolvers
The standard way to create a Promise object is to pass the appropriate callback to the constructor.
const myPromise = new Promise((resolve, reject) => {
/* Some code */
if(result) {
resolve("Ok")
} else {
reject(new Error("Rejected"))
}
})
We run into a problem when we want to handle Promise from outside the callback levels. This is, of course, possible, but requires writing a piece of not so nice code.
let resolve;
let reject;
const myPromise = new Promise((resolve_, reject_) => {
resolve = resolve_;
reject = reject_;
})
/* Some code */
if(result) {
resolve("Ok")
} else {
reject(new Error("Rejected"))
}
The newly proposed API is expected to offer a clearer solution to this problem.
const { promise, resolve, reject } = Promise.withResolvers();
/* Some code */
if(result) {
resolve("Ok")
} else {
reject(new Error("Rejected"))
}
TC39 Proposal – Promise.withResolvers
3. Generic Components are coming to Vue
Vue 3.3 is just around the corner and information about the features heading to the framework is slowly starting appear here and there. This week Evan You revealed that generic components will be the part of the next release. This is one of the longer-awaited Vue functionalities, as the first discussions about it took place back in 2021!
<script
setup
lang="ts"
generic="Clearable extends boolean, ValueType extends string | number | null | undefined"
>
defineProps<{
clearable?: Clearable;
value?: ValueType;;
}>
</script>
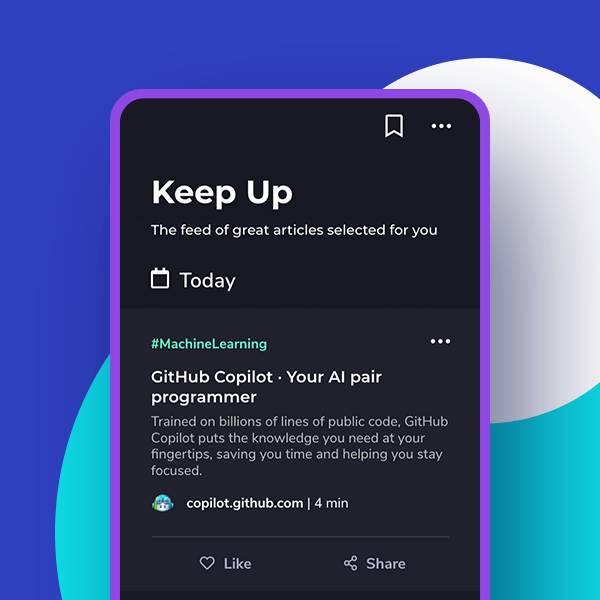
4. Microsoft has migrated Teams to WebView2
To conclude today’s review, we have a Case Study from Microsoft, which has just completed the migration of Microsoft Teams from AngularJS to React and from Electron to WebView2. As a reminder, WebView2 is a technology that enables embedding in native Microsoft Edge applications. What React and AngularJS are, we probably don’t need to remind anyone.
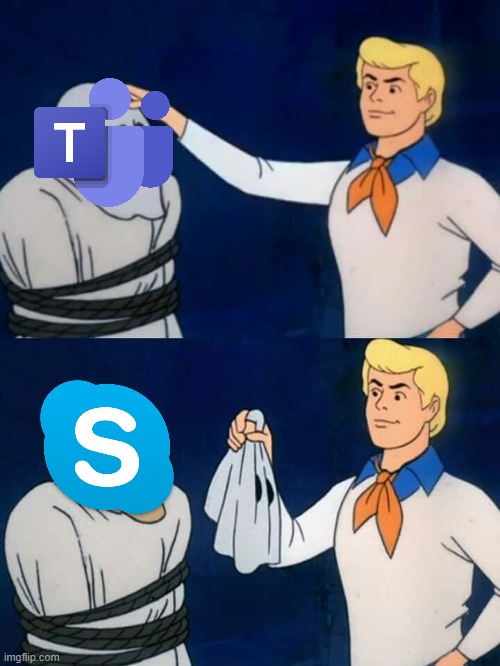
The migration brought significant improvements in both memory and CPU usage. The updated version has already started to hit “regular customers,” and will soon begin to hit commercial ones as well. If you’re curious about what the Microsoft Teams architecture looks like now, and where it managed to get the most bang for the buck, you can learn all about it from the note linked below.